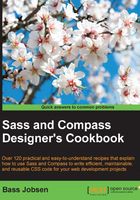
Using the @debug, @warn, and @error directives
In this recipe, you will learn how to use the @debug
, @warn
, and @error
directives to debug your code or validate the input for mixins and functions.
Getting ready
This recipe requires the command-line Ruby Sass compiler installed. In the Installing Sass for command-line usage recipe of Chapter 1, Getting Started with Sass, you can read about how to install Ruby and Ruby Sass. You can edit the Sass templates with your favorite text editor.
How to do it...
Use the following steps to learn how to use the @debug
, @warn
, and @error
directives to debug your Sass code:
- Create a Sass file
test.scss
that contains the following SCSS code:@mixin set-width($width) { @if $width < 50 { @error "width should be >= 50"; $width: 1px * $width; } @if unit($width) not 'px' { @warn "width (#{$width}) converted to pixels"; $width: 1px * $width; } $width: $width * 10; @debug "width: #{$width}"; width: $width; } div { @include set-width(50); }
- Then, run the following command in your console:
sass test.scss test.css
- You will find that the preceding command creates both a
test.css
and atest.css.map
file. In your console, you should find the following output:WARNING: width (50) converted to pixels on line 9 of test.scss, in `set-width' from line 19 of test.scss test.scss:14 DEBUG: width: 500px
- Finally, change
@include set-width(50);
into@include set-width(49);
in thetest.scss
file and run the command from the second step again. - Now, the output to the console will look as follows:
Error: width should be >= 50 on line 4 of test.scss, in `set-width' from line 19 of test.scss Use --trace for backtrace.
How it works...
SassScript is a small set of extensions used by Sass in addition to the plain CSS property syntax. SassScript enables you to use variables, arithmetic, and extra functions to set the values of your CSS properties. You can also use SassScript to set property and selector names via interpolation (refer to Chapter 3, Variables, Mixins, and Functions). In the Using Sass interactive mode and SassScript recipe of Chapter 1, Getting Started with Sass, you can read how to test SassScript by running Sass in the interactive mode.
The @debug
, @warn
, and @error
directives enable you to write SassScript expressions to the standard error output stream. You can use this information to debug your code or verify the correct usage of reusable and complex code, such as mixins and functions. You can read more about mixins and the @function
directive in Chapter 3, Variables, Mixins and Functions of this book, and Sass the @if
directive is described in the Using @if recipe of Chapter 8, Advanced Sass Coding.
The @debug
and @warn
directives only write a message to the output stream. The @error
directive generates an error, just like a normal Sass error and stops the compilation process.
There's more...
The output of the @debug
and @warn
directives can be suppressed by using the -quiet
option. You can repeat the command from the second step of this recipe with the -quiet
option by writing the following command in your console:
sass --quiet test.scss test.css
The preceding command compiles your Sass code without generating any output to the standard error output stream. The @error
directive cannot be suppressed or ignored. Although the @error
directive stops the compilation process, Sass does generate the test.css
file. After an error is thrown by the @error
directive, the test.css
file does not contain valid CSS code; instead, the error trace is saved to it. A test.css.map
source map file will not be generated.