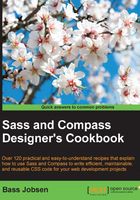
Using CSS source maps to debug your code
Most Sass projects merge and compile code from multiple source files into a single CSS file. This CSS file has also been minified in most cases. When you are inspecting the source of CSS files with the developer tools of your browser, you cannot relate the style effects to your original Sass code. CSS source maps solve this problem by mapping the combined/minified file back to its unbuilt state.
Getting ready
This recipe requires only the Ruby Sass compiler to be installed. Read the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, to find out how to install the Ruby Sass compiler. Use a command-line editor, such as VIM, to edit your Sass files. Refer to the Writing our code in a text editor recipe of Chapter 1, Getting Started with Sass, to read more about editing your Sass files. Finally, you will need a modern browser with support for the source map protocol. Both Google Chrome and Firefox support source maps debugging for a while. Also, MS Internet Explorer version 11 and up support version 3 of the source map protocol.
How to do it...
Use the step beneath to find out how to use CSS source maps to debug your Sass code:
- Create a file structure, as shown in the following image:
The
main.scss
file should only contain the following lines of code:@import 'components/header';
- In the
components/_headers.scss
file, type the SCSS code, as follows:// CSS RESET body, html { margin: 0; padding: 0; } @mixin header-styles($background-color) { background-color: $background-color; border: 3px solid darken($background-color, 20%); color: $header-default-font-color; } $header-margin: 10px !default; $header-childs-padding: 10px !default; $header-default-color: lightyellow !default; $header-default-font-color: tomato !default; .header { margin: $header-margin; * { padding: $header-childs-padding; } } .header-default { @include header-styles($header-default-color); }
- Now run the following command in your console:
sass sass/main.scss css/main.css
- Finally, create an
index.html
file and use thelink
attribute in thehead
section of this file to link the compiledcss/main.css
file. You can use the following code to link the style sheet:<link rel="stylesheet" type="text/css" href="css/main.css" />
The complete HTML code of
index.html
should look as follows:<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Sass with CSS source maps</title> <meta name="description" content="Sass with CSS source maps"> <meta name="author" content="Bass Jobsen"> <link rel="stylesheet" type="text/css" href="css/main.css" /> </head> <body> <header class="header header-default"> <h1>Write and debug Sass in brower</h1> </header> </body> </html>
- Open
index.html
from the previous step in your browser and open Developer Tools by right-clicking on the page and choosing the Inspect element option. You don't have to run a local web server, you can also open the file by using thefile:// protocol
. - Then, select
<header class="header header-default">
in the Elements tabs and you will find that the style rules for the header element are indeed associated with your_headers.scss
file instead of themain.css
file. The following figure will show you that the.header-default
style rules are defined in the_headers.scss
file in line 25:
How it works...
The sass sass/main.scss css/main.css
command not only creates css/main.css
, but also a css/main.css.map
file. The main.css.map
source map file contains the mapping for each selector and the style rule in the main.css
file. The map itself is stored in a base64 encoded-string and the source map file is in the JSON format.
In the beginning, source maps were introduced to map minify JavaScript to its origin source. Since version 3 of the source map protocol, support for CSS has been added. Sass generates the CSS source maps, as described previously, by default and adds a reference into the compiled CSS file, as follows:
/*# sourceMappingURL=main.css.map */
The web browser's developers tools use the preceding comment to read the source map and connect the style rules to their origin.
The components/_header-styleseaders.scss
file in this recipe is a so-called partial file. You can read more about partials in the Working with partials recipe of Chapter 1, Getting Started with Sass. This partial file contains some Sass code for this demo that you might not be familiar with. The code uses variables and mixins, which are both discussed in Chapter 3, Variables, Mixins, and Functions, of this book. Variables in SCSS start with a $
sign, and mixins are defined with the @mixin
directive and called with the @include
directive.
There's more...
In the last figure of the recipe, you found that the Sass source files are hyperlinked. Clicking on these links will take you to the source in the style editor, provided that the source files are reachable for the browser. In the Editing and debugging your Sass code in a browser recipe of this chapter, you can read how to use these features to edit and debug your Sass code.
The default --sourcemap=auto
setting sets relative paths where possible and file URIs elsewhere. Alternatively, you can use the --sourcemap=file
setting that forces the use of absolute file URIs always. The other settings are --sourcemap=none
which completely disables sources maps, and the --sourcemap=inline
setting which includes the source text in the source map.
In the past, people used the FireSass Firebug plugin together with the --debug
option to find the Sass code source for a certain style rule. Because Sass, Compass, and all the major browsers support source maps now, you should not use the --debug
option any more.
Both Sass and Compass also support the --line-comments
option; this option emits comments in the generated CSS, indicating the corresponding source line. These comments will look like that shown in the example CSS code here:
/* line 18, components/_header.scss */ .header { margin: 10px; } /* line 20, components/_header.scss */ .header * { padding: 10px; }
You can use these line comments when compiling your code on the command line or debugging your code without a browser. In the Commenting your code in SCSS syntax recipe of this chapter, you can read how comments in Sass compile into your final CSS code.
See also
The Source Map Revision 3 proposal can be found at https://docs.google.com/a/google.com/document/d/1U1RGAehQwRypUTovF1KRlpiOFze0b-_2gc6fAH0KY0k/. This proposal has been implemented by the Sass compiler, other preprocessors, and all the major browsers.