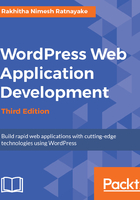
Handling registration form submission
Once the user fills in the data and clicks the Submit button, we have to execute quite a few tasks in order to register a user in the WordPress database. Let's figure out the main tasks for registering a user:
- Validating form data
- Registering the user details
- Creating and saving the activation code
- Sending e-mail notifications with an activate link or redirecting the user for payments based on the selected user type
We will be using a separate function called register_user to handle form submission. Validating user data is one of the main tasks in form submission handling. So, let's define the register_user function using the init action inside the class constructer, as shown in the following code:
class WPWAF_Registration{
public function __construct(){
add_action( 'init', array( $this, 'register_user' ) );
}
}
Now let's take a look at the implementation of the register_user function inside the WPWAF_Registration class:
public function register_user(){
global $wpwaf_registration_params,$wpwaf_login_params;
......
if ($_POST['wpwaf_reg_submit'] ) {
$errors = array();
$user_login = ( isset ( $_POST['wpwaf_user'] ) ? $_POST['wpwaf_user'] : '' );
$user_email = ( isset ( $_POST['wpwaf_email'] ) ? $_POST['wpwaf_email'] : '' );
$user_type = ( isset ( $_POST['wpwaf_user_type'] ) ? $_POST['wpwaf_user_type'] : '' );
if ( empty( $user_login ) )
array_push( $errors, __('Please enter a username.','wpwaf') );
if ( empty( $user_email ) )
array_push( $errors, __('Please enter e-mail.','wpwaf') );
if ( empty( $user_type ) )
array_push( $errors, __('Please enter user type.','wpwaf') );
// Saving user details
// Including the template
}
The following steps are to be performed:
- First, we will check whether the request is made as POST.
- Then, we get the form data from the POST array.
- Finally, we will check the passed values for empty conditions and push the error messages to the $errors variable created at the beginning of this function.
Now, we can move into more advanced validations inside the register_user function, as shown in the following code:
$sanitized_user_login = sanitize_user( $user_login );
if ( !empty($user_email) && !is_email( $user_email ) )
array_push( $errors, __('Please enter valid email.','wpwaf'));
elseif ( email_exists( $user_email ) )
array_push( $errors, __('User with this email already registered.','wpwaf'));
if ( empty( $sanitized_user_login ) || !validate_username( $user_login ) )
array_push( $errors, __('Invalid username.','wpwaf') );
elseif ( username_exists( $sanitized_user_login ) )
array_push( $errors, __('Username already exists.','wpwaf') );
The steps to perform are as follows:
- First, we will use the existing sanitize_user function and remove unsafe characters from the username.
- Then, we will make validations on the e-mail to check whether it's valid and its existence status in the system. Both the email_exists and username_exists functions check for the existence of an email and username from the database. Once all the validations are completed, the errors array will be either empty or filled with error messages.
Next, we need to pass the registration form data and errors to the registration form template. In the beginning of this function, we added a global variable called $wpwaf_registration_params to keep data for templates. So we can assign the errors and data to this variable, as shown in the following code:
$wpwaf_registration_params['errors'] = $errors;
$wpwaf_registration_params['user_login'] = $user_login;
$wpwaf_registration_params['user_email'] = $user_email;
$wpwaf_registration_params['user_type'] = $user_type;
We added $wpwaf_registration_params as a global variable in the start of this section while creating the registration template. If we get validation errors in the form, we can directly print the contents of the error array on top of the form using the $wpwaf_registration_params variable as shown in the registration template. Here is a preview of our registration screen with generated error messages:

Also, it's important to repopulate the form values once errors are generated. We have passed the user-submitted data to the template using the $wpwaf_registration_params variable. Therefore, we can directly access the POST variables inside the template to echo the values, as shown in the updated registration form:
<form id='wpwaf-registration-form' method='post' action='<?php echo get_site_url() . '/user/register'; ?>'>
<ul>
<li>
<label class='wpwaf_frm_label'><?php echo __('Username','wpwaf'); ?></label>
<input class='wpwaf_frm_field' type='text' id='wpwaf_user' name='wpwaf_user' value='<?php echo isset( $user_login ) ? $user_login : ''; ?>' />
</li>
<li>
<label class='wpwaf_frm_label'><?php echo __('E- mail','wpwaf'); ?></label>
<input class='wpwaf_frm_field' type='text' id='wpwaf_email' name='wpwaf_email' value='<?php echo isset( $user_email ) ? $user_email : ''; ?>' />
</li>
<li>
<label class='wpwaf_frm_label'><?php echo __('User Type','wpwaf'); ?></label>
<select class='wpwaf_frm_field' name='wpwaf_user_type'>
<option <?php echo (isset( $user_type ) && $user_type == 'wpwaf_premium_member') ? 'selected' : ''; ?> value='wpwaf_premium_member'><?php echo __('Premium Member','wpwaf'); ?></option>
<option <?php echo (isset( $user_type ) && $user_type == 'wpwaf_free_member') ? 'selected' : ''; ?> value='wpwaf_free_member'> <?php echo __('Free Member','wpwaf'); ?></option>
</select>
</li>
<li>
<label class='wpwaf_frm_label' for=''> </label>
<input type='submit' name='wpwaf_reg_submit' value='<?php echo __('Register','wpwaf'); ?>' />
</li>
</ul>
</form>