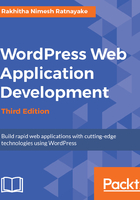
Preparing the plugin
As developers, we have the option to build a complete application with standalone plugins or use various independent plugins to cater to specific modules. Generally, many developers tend to use a bunch of existing plugins without developing their own. The main reason behind using other plugins is that developers want to save time and cost when developing WordPress websites. However, in most scenarios, this process is not ideal for complex web application development. I recommend that you select fewer plugins and improve them with additional functionality or develop everything from scratch.
Throughout this book, we will be integrating all the independent modules into a standalone plugin. We will create a specific plugin for our forum management application. So, let's get started by creating a new folder called wpwa-forum, inside the /wp-content/plugins folder.
Then, create a PHP file inside the folder and save it as wpwa-forum.php. Now, it's time to add the plugin definition, as shown in the following code:
<?php
/*
Plugin Name: WPWAF Forum
Plugin URI : -
Description: Forum Management application for WordPress Web Application Development 3rd Edition
Version : 1.0
Author : Rakhitha Nimesh
Author URI: http://www.wpexpertdeveloper.com/
*/
In the previous chapter, we created a plugin with a specific initial structure. We will be using the same coding structure for the forum plugin. Let's look at the basic structure of the wpwa-forum.php file using the following code:
if( !class_exists( 'WPWAF_Forum' ) ) {
class WPWAF_Forum{
private static $instance;
public static function instance() {
if ( ! isset( self::$instance ) && ! ( self::$instance instanceof WPWAF_Forum ) ) {
self::$instance = new WPWAF_Forum();
self::$instance->setup_constants();
self::$instance->includes();
add_action( 'admin_enqueue_scripts',array(self::$instance,'load_admin_scripts'),9);
add_action( 'wp_enqueue_scripts',array(self::$instance,'load_scripts'),9);
// Class object intialization
}
return self::$instance;
}
public function setup_constants() {
if ( ! defined( 'WPWAF_VERSION' ) ) {
define( 'WPWAF_VERSION', '1.0' );
}
if ( ! defined( 'WPWAF_PLUGIN_DIR' ) ) {
define( 'WPWAF_PLUGIN_DIR', plugin_dir_path( __FILE__ ) );
}
if ( ! defined( 'WPWAF_PLUGIN_URL' ) ) {
define( 'WPWAF_PLUGIN_URL', plugin_dir_url( __FILE__ ) );
}
}
public function load_scripts(){ }
public function load_admin_scripts(){ }
private function includes() { }
public function load_textdomain() {}
}
}
Once the class is defined, we can initialize the class using the following code as we did previously in the question-answer plugin:
function WPWAF_Forum() {
global $wpwaf;
$wpwaf = WPWAF_Forum::instance();
}
WPWAF_Forum();
The important aspect of this structure is the use of the singleton design pattern which creates only one instance of the main class and returns it every time we need it. Let's look at the Wikipedia definition of the singleton pattern:
We can develop plugins without the singleton pattern. In such methods, you need to make sure that you don't initialize the main object multiple times, which results in execution of the same action hook multiple times. There are many plugins with this issue and it's extremely difficult to identify the conflicts since the actions are called multiple times. Using this structure will prevent accidental initialization of unnecessary classes.