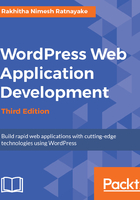
Changing the status of answers
Once the users provide their answers, the creator of the question should be able to mark them as correct or incorrect answers. So, we will implement a button for each answer to mark the status. Only the creator of the questions will be able to mark the answers. Once the button is clicked, an AJAX request will be made to store the status of the answer in the database.
Implementation of the comment_list function goes inside the main class of wpwa-questions.php file of our plugin. This function contains lengthy code, which is not necessary for our explanations. Hence, I'll be explaining the important sections of the code. It's ideal to work with the full code for the comment_list function from the source code folder:
function comment_list( $comment, $args, $depth ) {
global $post;
$GLOBALS['comment'] = $comment;
$current_user = wp_get_current_user();
$author_id = $post->post_author;
$show_answer_status = false;
if ( is_user_logged_in() && $current_user->ID == $author_id ){
$show_answer_status = true;
}
$comment_id = get_comment_ID();
$answer_status = get_comment_meta( $comment_id,
"_wpwa_answer_status", true );
// Rest of the Code
}
The comment_list function is used as the callback function of the comments list, and hence it will contain three parameters by default. Remember that the button for marking the answer status should be only visible to the creator of the question.
In order to change the status of answers, follow these steps:
- First, we will get the current logged-in user from the wp_get_current_user function. Also, we can get the creator of the question using the global $post object.
- Next, we will check whether the logged-in user created the question. If so, we will set show_answer_status to true. Also, we have to retrieve the status of the current answer by passing the comment_id and _wpwa_answer_status keys to the get_comment_meta function.
- Then, we will have to include the common code for generating a comments list with the necessary condition checks.
- Open the wpwa-questions.php file of the plugin and go through the rest of the comment_list function to get an idea of how the comments loop works.
- Next, we have to highlight the correct answers of each question and I'll be using an image as the highlighter. In the source code, we use the following code after the header tag to show the correct answer highlighter:
<?php
// Display image of a tick for correct answers
if ( $answer_status ) {
echo "<div class='tick'><img src='".plugins_url(
'img/tick.png', __FILE__ )."' alt='Answer Status'
/></div>";
}
?>
- In the source code, you will see a <div> element with the class reply for creating the comment reply link. We will need to insert our answer button status code right after this, as shown in the following code:
<div>
<?php
// Display the button for authors to make the answer as correct or incorrect
if ( $show_answer_status ) {
$question_status = '';
$question_status_text = '';
if ( $answer_status ) {
$question_status = 'invalid';
question_status_text = __('Mark as
Incorrect','wpwa_questions');
} else {
$question_status = 'valid';
$question_status_text = __('Mark as
Correct','wpwa_questions');
}
?>
<input type="button" value="<?php echo
$question_status_text; ?>" class="answer-status
answer_status-<?php echo $comment_id; ?>"
data-ques-status="<?php echo $question_status; ?>" />
<input type="hidden" value="<?php echo $comment_id; ?>"
class="hcomment" />
<?php
}
?>
</div>
- If the show_answer_status variable is set to true, we get the comment ID, which will be our answer ID, using the get_comment_ID function. Then, we will get the status of answer as true or false using the _wpwa_answer_status key from the wp_commentmeta table.
- Based on the returned value, we will define buttons for either Mark as Incorrect or Mark as Correct. Also, we will specify some CSS classes and HTML5 data attributes to be used later with jQuery.
- Finally, we keep the comment_id in a hidden variable called hcomment.
- Once you include the code, the button will be displayed for the author of the question, as shown in the following screen:

- Next, we need to implement the AJAX request for marking the status of the answer as true or false.
Before this, we need to see how we can include our scripts and styles into WordPress plugins. We added empty functions to include the scripts and styles while preparing the structure of this plugin. Here is the code for including custom scripts and styles for our plugin inside the load_scripts function we created earlier. Copy the following code into the load_scripts function of the wpwa-questions.php file of your plugin:
public function load_scripts() {
wp_enqueue_script( 'jquery' );
wp_register_script( 'wpwa-questions', plugins_url(
'js/questions.js', __FILE__ ), array('jquery'), '1.0', TRUE );
wp_enqueue_script( 'wpwa-questions' );
wp_register_style( 'wpwa-questions-css', plugins_url(
'css/questions.css', __FILE__ ) );
wp_enqueue_style( 'wpwa-questions-css' );
$config_array = array(
'ajaxURL' => admin_url( 'admin-ajax.php' ),
'ajaxNonce' => wp_create_nonce( 'ques-nonce' )
);
wp_localize_script( 'wpwa-questions', 'wpwaconf', $config_array
);
}
WordPress comes with an action hook built-in called wp_enqueue_scripts for adding JavaScript and CSS files. The wp_enqueue_script action is used to include script files into the page while the wp_register_script action is used to add custom files. Since jQuery is built-in to WordPress, we can just use the wp_enqueue_script action to include jQuery into the page. We also have a custom JavaScript file called questions.js, which will contain the functions for our application.
Inside JavaScript files, we cannot access the PHP variables directly. WordPress provides a function called wp_localize_script to pass PHP variables into script files. The first parameter contains the handle of the script for binding data, which will be wp_questions in this scenario. The second parameter is the variable name to be used inside JavaScript files to access these values. The third and final parameters will be the configuration array with the values.
Then, we can include our questions.css file using the wp_register_style and wp_enqueue_style functions, which will be similar to JavaScript, file inclusion syntax, we discussed previously. Now, everything is set up properly to create the AJAX request.