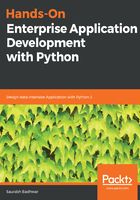
Method resolution order in multiple inheritance
So, based on the previous example, what happens if we create an object of the C class?
>>> Cobj = C()
Class C
As we can see, only the derived class constructor was called here. So what if we wanted to call the parent class constructor also? For that, we will require the help of the super() call inside our class C constructor. To see it in action, let's modify the implementation of C a little bit:
>>> class C(A,B):
... def __init__(self):
... print("C")
... super().__init__()
>>> Cobj = C()
C
A
As soon as we created the object of the derived class, we can see that the derived class constructor was called first, followed by the constructor of the first inherited class. The super() call automatically resolved to the first inherited class.
So, what if we wanted to call the constructors of all the classes in order? For that, we could have added a super call to all the classes:
class A:
def __init__(self):
print("Class A")
super().__init__()
class B:
def __init__(self):
print("Class B")
super().__init__()
class C(A,B):
def __init__(self):
print("Class C")
super().__init__()
>>> Cobj = C()
C
A
B
When we created an object of C, we can see that all the constructors are called in the order of how they were inherited. The first call went to the derived class constructor, followed by the call to constructor A, which came first in the inheritance chain, and then the call was made to constructor B.
Here in this example, everything seems to be fine because none of the constructors take any parameters in their initialization call. As soon as the constructors start taking some parameters in the initialization call, things start to get complicated. Now we need to make sure every constructor accepts all the parameters. Now, if we implement this approach, we have tightly coupled all the classes, which in itself defeats the purpose of inheritance where one class is supposed to do only one thing. To deal with these kinds of problems, we can use something known as a mixin.