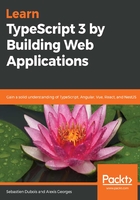
Creating the todo list with TypeScript arrays
Now we'll create our todo list in TypeScript, and then we'll implement a way to add new items to that list.
To model our todo list, we will make use of a TypeScript array.
Open todo-it.ts, then, after the console.log statement, add a new constant: const todoList: string[] = [];.
If you log the array object at this point, you'll see that the web browser console can help you peek into the values: console.log("Current todo list: ", todoList);.
Here's the corresponding output in the Chrome Developer Tools:
In TypeScript, our array is type safe because we have defined the type of data that it will hold. If you try to add another type of data into the array, for example, todoList.push(1);, then TypeScript will complain: Argument of type '1' is not assignable to parameter of type 'string'.
It is really important to understand that this safety only exists at compile time. Once the JavaScript version of the code executes in the web browser, those restrictions don't apply anymore, and TypeScript can't help you there. You can easily verify this now in your browser. In the console, type the following: todoList.push(1);. Did that fail? Of course not! If you look at the content of the todoList array now, you'll see that it contains the item, even though it isn't a string. Here's an example:
